In the ever-evolving landscape of web development, one term that frequently pops up is CORS, or Cross-Origin Resource Sharing. This mechanism is a crucial part of modern web applications, enabling them to request resources from different origins while maintaining security. As we delve into the world of CORS, we'll explore its relevance, how it works, and why it's so important in today's web development environment. We'll also discuss the Same-Origin Policy, a fundamental web security concept that CORS extends and enhances. By the end of this article, we'll have a comprehensive understanding of CORS, its role in web security, and how it impacts the way we build and interact with web applications. So, let's embark on this journey of understanding CORS, a critical component that bridges the gap between functionality and security in web development.
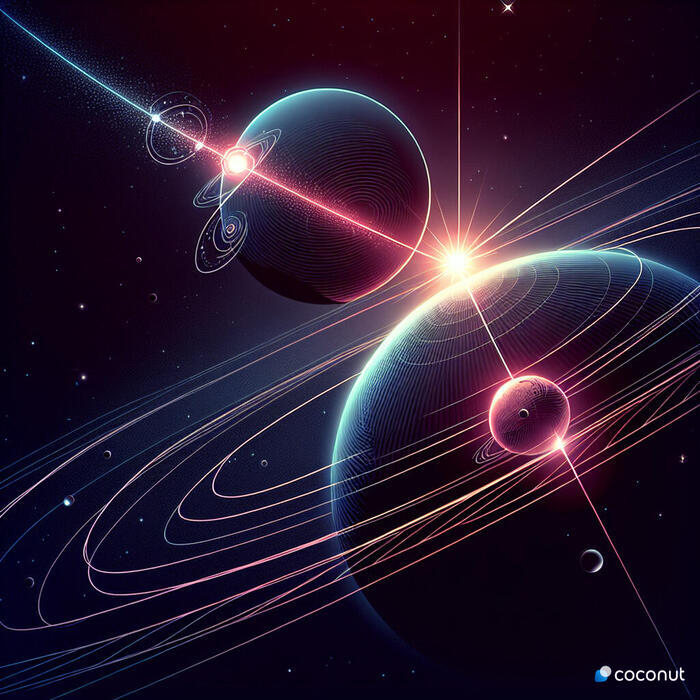
Understanding the Same-Origin Policy
Before we delve into CORS, it's essential to understand the Same-Origin Policy (SOP), a foundational concept in web security. The SOP is a critical security measure implemented by web browsers to prevent potentially malicious behavior. It restricts how a document or script loaded from one origin can interact with a resource from another origin. This policy is the cornerstone of web application security, preventing document or script from one origin from getting or setting properties of a document from another. By understanding the SOP, we can better appreciate the role of CORS and how it extends this policy to allow more flexible interactions between different origins while maintaining web security.
Definition and Purpose of Same-Origin Policy
The Same-Origin Policy is a security measure that restricts how a document or script loaded from one origin can interact with a resource from another origin. An origin is defined by the scheme (protocol), host (domain), and port of a URL. The SOP is crucial for web security as it prevents potentially malicious scripts on one page from obtaining access to sensitive data on another web page through that page's Document Object Model (DOM). This policy ensures that scripts cannot read data from another site just because the user visiting the site has access to it.
Limitations Imposed by Same-Origin Policy
While the Same-Origin Policy is a vital security measure, it imposes certain limitations on web resources. It restricts web pages from sharing resources unless they come from the same origin. This means that a web page cannot make requests to a different domain than the one it came from. This restriction can hinder the functionality of web applications that need to access APIs or resources hosted on different domains. It's in overcoming these limitations that CORS comes into play, providing a secure way for web applications to access cross-origin resources.
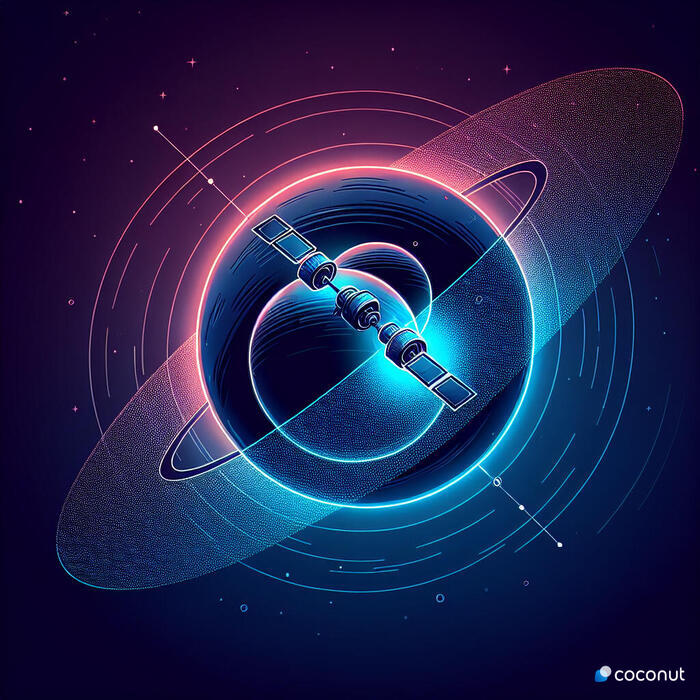
The Mechanics of CORS
Now that we understand the Same-Origin Policy and its limitations, let's delve into the mechanics of CORS. CORS is a mechanism that uses additional HTTP headers to tell browsers to give a web application running at one origin, access to selected resources from a different origin. A web application makes a cross-origin HTTP request when it requests a resource from a different domain, protocol, or port than the one from which the current document originated. The CORS mechanism supports secure cross-origin requests and data transfers between browsers and servers. Modern browsers use CORS in an API container - such as XMLHttpRequest or Fetch - to mitigate risks of cross-origin HTTP requests. The process begins with the browser sending an HTTP OPTIONS request method to the server to discover whether the server will accept its request, based on the origin. This is known as a preflight request. The server then responds with Access-Control headers to indicate which origins, methods, and headers are allowed. If the server allows the request, the actual request is sent, and the server includes the Access-Control-Allow-Origin header in its response to indicate that the origin has permission to access the resource. It's important to note that CORS is not a security vulnerability, but a standard that was introduced to allow secure cross-origin data transfers. It provides a framework that enables controlled access to resources located outside of a given domain, instead of arbitrary access, thereby enhancing web application security.
How CORS Extends the Same-Origin Policy
CORS extends the Same-Origin Policy by adding new HTTP headers that allow servers to describe the set of origins that are permitted to read that information using a web browser. Instead of blocking all cross-origin requests, CORS gives servers the flexibility to decide whether to authorize requests based on the origin. This means that with CORS, web applications can make cross-origin requests, which the Same-Origin Policy would otherwise prohibit, while maintaining security. CORS essentially provides a secure method to loosen the restrictions of the Same-Origin Policy, enabling more complex web applications while still maintaining the security foundations that the Same-Origin Policy provides. In essence, CORS is a compromise between the robust security provided by the Same-Origin Policy and the practical need for cross-origin requests in modern web applications. It's a testament to the evolving nature of web development, where security and functionality must go hand in hand.
CORS HTTP Headers Explained
Cross-Origin Resource Sharing (CORS) is a mechanism that uses additional HTTP headers to tell browsers to give a web application running at one origin, access to selected resources from a different origin. A web application executes a cross-origin HTTP request when it requests a resource from a different domain, protocol, or port than the one from which the current document originated. There are several HTTP headers related to CORS that serve different functions. The 'Access-Control-Allow-Origin' header determines which origins are allowed to read the response. It can either be a specific origin or a wildcard '*' for unrestricted access. The 'Access-Control-Allow-Credentials' header indicates whether the response to the request can be exposed when the credentials flag is true. The 'Access-Control-Allow-Methods' header specifies the method or methods allowed when accessing the resource. This is used in response to a preflight request. The 'Access-Control-Allow-Headers' header is used in response to a preflight request to indicate which HTTP headers can be used during the actual request. The 'Access-Control-Expose-Headers' header lets a server whitelist headers that browsers are allowed to access. The 'Access-Control-Max-Age' header indicates how long the results of a preflight request can be cached. Lastly, the 'Access-Control-Request-Method', 'Access-Control-Request-Headers', and 'Origin' headers are used when issuing a preflight request to the server.

Browser's Role in CORS
Browsers play a crucial role in implementing CORS and managing cross-origin requests. When a web application attempts to access resources from a different origin, the browser automatically sends a CORS preflight request to the server hosting the resources. This preflight request is an HTTP OPTIONS request method, and it includes headers like 'Origin', 'Access-Control-Request-Method', and 'Access-Control-Request-Headers' to inform the server about the origin that is making the request and the type of request being made.The server then responds to the preflight request with CORS headers that indicate whether the original request is allowed. If the server allows the request, the browser then sends the original request. If the server does not allow the request, the browser blocks the request and returns a CORS error. This process is transparent to the user and is handled entirely by the browser and the server. The browser's role in CORS is essentially to enforce the server's access controls and ensure that web applications cannot make cross-origin requests that the server has not authorized.
Setting Up CORS on the Server
Setting up CORS on the server involves configuring the server to respond to CORS preflight requests with the appropriate CORS headers. This configuration varies depending on the type of server. For an Apache server, you can set up CORS by adding the necessary 'Header set' directives to the server's .htaccess file or to the virtual host file. For example, to allow all origins, you would add the directive 'Header set Access-Control-Allow-Origin "*"'.For an Nginx server, you can set up CORS by adding 'add_header' directives to the server block in the server's configuration file. For example, to allow all origins, you would add the directive 'add_header 'Access-Control-Allow-Origin *';'.For a Node.js server, you can set up CORS by using the cors middleware package for Express.js. After installing the cors package with npm, you can use it in your application like this: 'var express = require('express'); var cors = require('cors'); var app = express(); app.use(cors());'.In all cases, you should replace the wildcard '*' with the specific origin or origins that you want to allow if you want to restrict access to specific origins. You can also add other CORS headers as needed to control which methods and headers are allowed, whether credentials are allowed, and other aspects of CORS.
Configuring CORS in Apache, Nginx, and Node.js
Configuring CORS in Apache, Nginx, and Node.js involves adding specific directives or middleware to your server's configuration. In Apache, you can add the following lines to your .htaccess file to enable CORS: 'Header set Access-Control-Allow-Origin "*"' and 'Header set Access-Control-Allow-Methods "GET, POST, OPTIONS"'.In Nginx, you can add the following lines to your server block to enable CORS: 'add_header 'Access-Control-Allow-Origin *';' and 'add_header 'Access-Control-Allow-Methods GET, POST, OPTIONS;';'.In Node.js, you can use the cors middleware package for Express.js to enable CORS. First, install the cors package with npm: 'npm install cors'. Then, use it in your application like this: 'var express = require('express'); var cors = require('cors'); var app = express(); app.use(cors());'.Remember to replace the wildcard '*' with the specific origin or origins that you want to allow if you want to restrict access to specific origins. You can also add other CORS headers as needed to control which methods and headers are allowed, whether credentials are allowed, and other aspects of CORS.
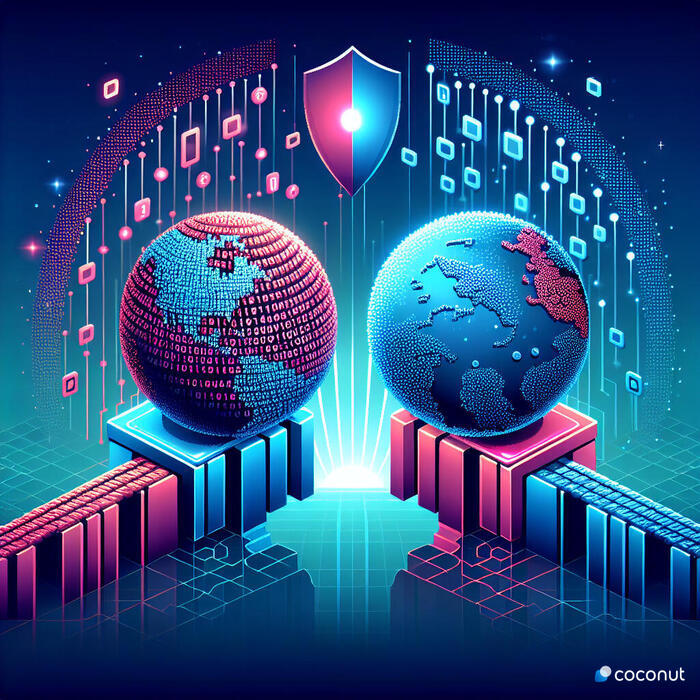
Handling CORS in Cloud Services and CDNs
Managing CORS configurations in cloud-based services and content delivery networks (CDNs) can be a bit different from managing them on a traditional server. In cloud services like AWS, you can configure CORS by adding CORS configuration to the bucket policy or the CORS configuration of the S3 bucket. The CORS configuration is an XML document that specifies which origins are allowed, which methods are allowed, and other CORS settings.In CDNs like Cloudflare, you can configure CORS by creating a page rule that adds the necessary CORS headers. The page rule should match the URL pattern of the resources that you want to apply CORS to, and it should include settings to add the 'Access-Control-Allow-Origin' and other CORS headers.In both cases, you should replace the wildcard '*' with the specific origin or origins that you want to allow if you want to restrict access to specific origins. You can also add other CORS headers as needed to control which methods and headers are allowed, whether credentials are allowed, and other aspects of CORS.
Common CORS Scenarios and Solutions
CORS issues can arise in a variety of scenarios, and understanding these scenarios can help you troubleshoot and resolve CORS issues more effectively. One common scenario is when a web application tries to make a cross-origin request without the server's permission. In this case, the browser blocks the request and returns a CORS error. The solution is to configure the server to respond to CORS preflight requests with the appropriate CORS headers that allow the request.Another common scenario is when a web application tries to make a cross-origin request with credentials, but the server does not allow credentials. In this case, the browser blocks the request and returns a CORS error. The solution is to configure the server to respond to CORS preflight requests with the 'Access-Control-Allow-Credentials' header set to 'true'.Yet another common scenario is when a web application tries to make a cross-origin request with a method or header that the server does not allow. In this case, the browser blocks the request and returns a CORS error. The solution is to configure the server to respond to CORS preflight requests with the 'Access-Control-Allow-Methods' and 'Access-Control-Allow-Headers' headers that allow the method or header.In all these scenarios, the key to resolving the CORS issue is to correctly configure the server to respond to CORS preflight requests with the appropriate CORS headers.
Debugging CORS Errors
Debugging CORS errors can be challenging, but there are several tools and techniques that can help. One of the most useful tools for debugging CORS errors is the browser's developer tools. The network tab in the developer tools shows all the network requests that the web page makes, including the CORS preflight requests and the server's responses. By examining the headers of the preflight requests and the responses, you can often identify what is causing the CORS error.Another useful tool for debugging CORS errors is curl, a command-line tool for making HTTP requests. You can use curl to manually send a CORS preflight request to the server and examine the server's response. This can help you identify whether the server is correctly configured to respond to CORS preflight requests.In addition to these tools, understanding the CORS mechanism and the role of the different CORS headers can also help you debug CORS errors. By understanding how CORS works, you can better understand what is causing a CORS error and how to fix it.
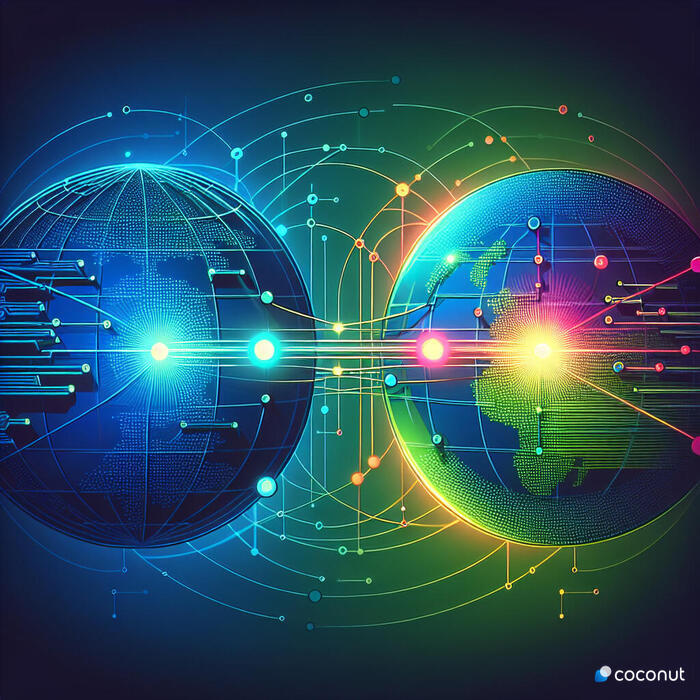
Best Practices for Managing CORS
Managing CORS effectively requires following some best practices. First, be specific about which origins you allow. While it may be tempting to use the wildcard '*' to allow all origins, this can be a security risk. It's better to specify the exact origins that you want to allow.Second, be specific about which methods and headers you allow. Again, it may be tempting to allow all methods and headers, but this can also be a security risk. It's better to specify the exact methods and headers that you want to allow.Third, use credentials sparingly. Allowing credentials can be a security risk, so only allow credentials if absolutely necessary.Fourth, use the 'Access-Control-Max-Age' header to cache preflight responses. This can improve performance by reducing the number of preflight requests.Finally, monitor and debug CORS issues regularly. Use the browser's developer tools and other tools to monitor CORS requests and responses, and debug any CORS errors that occur.
Security Implications of CORS
Cross-Origin Resource Sharing (CORS) is a fundamental aspect of modern web development, enabling resources to be shared across different origins. However, it also presents a range of security considerations that we must be aware of. The primary security risk associated with CORS is that it can potentially allow malicious websites to access sensitive data. This is because CORS relaxes the same-origin policy, a security measure that restricts how a document or script loaded from one origin can interact with a resource from another origin. By enabling CORS, we are essentially allowing a website to make a cross-origin request, potentially exposing sensitive data to malicious actors. Another security risk associated with CORS is that it can be used to bypass standard security measures. For instance, an attacker could use CORS to make a request to a website that would otherwise be blocked by the browser's same-origin policy. This could potentially allow the attacker to access sensitive information or perform actions on the website without the user's knowledge or consent. Furthermore, CORS can also be exploited to perform cross-site request forgery (CSRF) attacks. In a CSRF attack, an attacker tricks a victim into performing an action on a website that the victim is authenticated on. This could potentially allow the attacker to perform actions on the website as the victim, potentially leading to unauthorized access or data loss. In addition, CORS can also be used to perform cross-site scripting (XSS) attacks. In an XSS attack, an attacker injects malicious scripts into web pages viewed by other users. These scripts can then be used to steal sensitive information, such as login credentials or personal data. Finally, CORS can also be used to perform server-side request forgery (SSRF) attacks. In an SSRF attack, an attacker can make requests to internal resources that are not normally accessible from the outside. This could potentially allow the attacker to access sensitive information or perform actions on internal systems. In conclusion, while CORS is a powerful tool that can greatly enhance the functionality of a website, it also presents a range of security risks that must be carefully managed.
Risks of Misconfigured CORS
Misconfigured CORS settings can pose significant security risks. If CORS is not properly configured, it can potentially allow unauthorized access to sensitive data. For instance, if a website's CORS policy is too permissive, it could allow any website to make cross-origin requests, potentially exposing sensitive data to malicious actors. Moreover, misconfigured CORS settings can also be exploited to perform various types of attacks, such as CSRF, XSS, and SSRF attacks. For instance, if a website's CORS policy allows any website to make cross-origin requests with credentials, an attacker could potentially use this to perform a CSRF attack, tricking a victim into performing an action on the website without their knowledge or consent. Furthermore, misconfigured CORS settings can also lead to data leakage. For instance, if a website's CORS policy allows any website to read the response of a cross-origin request, an attacker could potentially use this to access sensitive data. In conclusion, misconfigured CORS settings can pose significant security risks, potentially allowing unauthorized access to sensitive data and enabling various types of attacks. Therefore, it is crucial to properly configure CORS settings to ensure the security of a website.
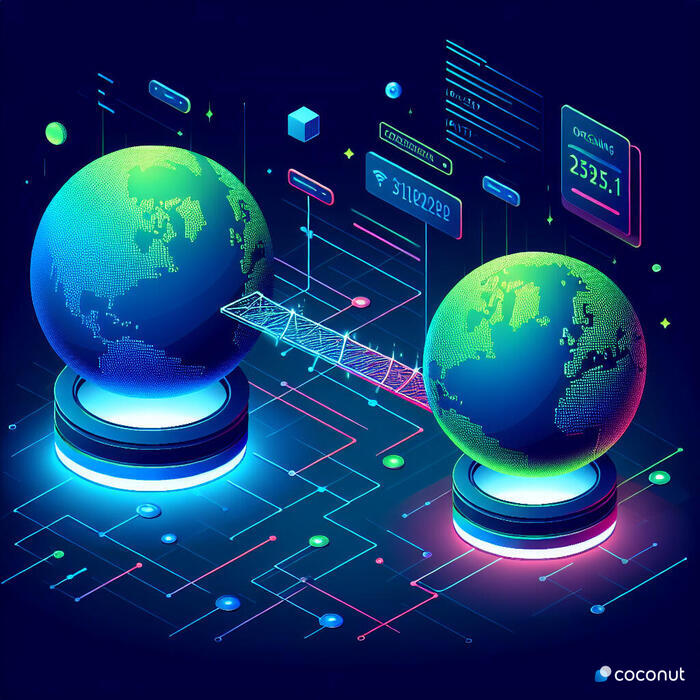
Ensuring Secure CORS Practices
To ensure secure CORS practices, it is crucial to properly configure CORS settings. This involves setting a strict CORS policy that only allows trusted websites to make cross-origin requests. This can help prevent unauthorized access to sensitive data and mitigate the risk of various types of attacks. Moreover, it is also important to regularly review and update CORS settings to ensure that they remain secure. This involves regularly checking for any changes in the trusted websites and updating the CORS policy accordingly. Furthermore, it is also crucial to implement additional security measures to complement CORS. This includes implementing a strong Content Security Policy (CSP) to prevent XSS attacks, and using anti-CSRF tokens to prevent CSRF attacks. In addition, it is also important to educate users about the risks associated with CORS and encourage them to practice safe browsing habits. This includes advising users to only visit trusted websites and to regularly update their browsers to ensure that they have the latest security updates. In conclusion, ensuring secure CORS practices involves properly configuring CORS settings, implementing additional security measures, and educating users about the risks associated with CORS. By doing so, we can help ensure the security of our website and protect our users' data.
Further Readings and Online Resources
To further explore the topic of CORS and its security implications, there are numerous online resources available. These resources provide in-depth information on the technical and security aspects of CORS, and offer insights into the latest trends and changes in web security and CORS adoption. For instance, there are numerous in-depth articles that explore the technical and security aspects of CORS in detail. These articles provide a comprehensive overview of CORS, explaining its mechanics, its benefits, and its potential security risks. They also offer practical advice on how to properly configure CORS settings to ensure the security of a website. In addition, there are also numerous online resources that present the latest statistics related to web security and CORS adoption. These resources provide valuable insights into the current state of web security, highlighting the latest trends and changes in CORS adoption. They also offer predictions on the future of web security, providing a glimpse into what we can expect in the coming years. Furthermore, there are also numerous studies and research papers that provide empirical evidence or new findings on CORS and web security. These studies offer valuable insights into the real-world implications of CORS, providing empirical evidence of its benefits and potential risks. They also offer new findings on CORS and web security, contributing to our understanding of these complex topics. In conclusion, there are numerous online resources available that can help us further explore the topic of CORS and its security implications. By taking advantage of these resources, we can deepen our understanding of CORS, stay updated on the latest trends and changes in web security, and ensure the security of our website.