React Native has become a popular choice for cross-platform mobile app development. It's a JavaScript framework that allows developers to build natively rendered mobile applications for iOS and Android. The beauty of React Native is that it enables you to write code once and deploy it on multiple platforms, saving time and resources. Now, when it comes to enhancing user engagement, video playback plays a significant role. Videos are a powerful medium to convey messages, tell stories, and engage users. They can make your app more interactive and visually appealing. Whether it's a social media app, an e-learning platform, or a streaming service, integrating a video player can significantly improve the user experience. In this article, we will guide you through the process of implementing a video player in your React Native application. We will discuss the selection and installation of a suitable library, setting up the video player, specifying the video source, enabling playback controls, and styling the video player for a better user interface. Let's dive in!
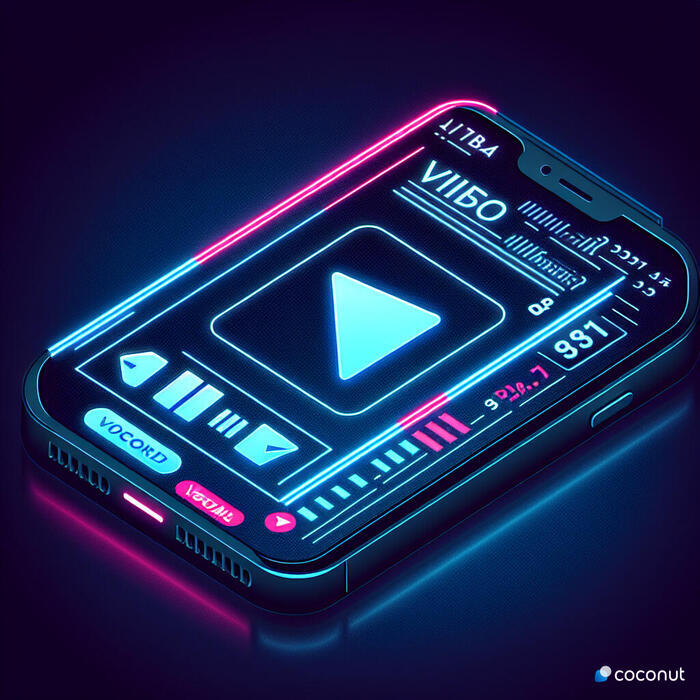
Getting Started with React Native Video Player
Selecting and Installing a Library
The first step in implementing a video player in React Native is selecting and installing a suitable library. There are several libraries available, each with its own set of features and capabilities. Some popular choices include react-native-video, react-native-video-player, and expo-av. When choosing a library, consider factors such as ease of use, customization options, support for different video formats, and compatibility with your project requirements. Once you've selected a library, you can install it using npm or yarn. For instance, if you choose react-native-video, you can install it by running the command 'npm install --save react-native-video' or 'yarn add react-native-video' in your project directory. After installation, you may need to link the library to your project using the 'react-native link' command. However, if you're using React Native 0.60 or higher, the library should be linked automatically thanks to React Native's auto-linking feature.
Setting Up the Video Player
Once the library is installed and linked, we can start setting up the video player. First, we need to import the necessary components from the library. For example, if we're using react-native-video, we would import the Video component like so: 'import Video from 'react-native-video''. Next, we render the Video component in our application. We can do this by including the Video component in our render method and specifying the necessary props. The Video component accepts several props that allow us to customize the video player's behavior and appearance. For instance, we can use the 'source' prop to specify the video source, the 'controls' prop to enable or disable playback controls, and the 'style' prop to apply custom styles to the video player. We'll discuss these props in more detail in the following sections.
Specifying the Video Source
Now that we have our video player set up, we need to specify the video source. The video source can be a local file or a remote URL. To specify a local video source, we need to require the video file in our project. For example, if we have a video file named 'my-video.mp4' in our project directory, we can set it as the video source like so: 'source={require('./my-video.mp4')}'. To specify a remote video source, we can pass a URL to the 'source' prop. For instance, 'source={{uri: 'https://example.com/my-video.mp4'}}'. It's important to note that the video file must be in a format that's supported by both iOS and Android. Commonly supported formats include MP4, M4V, and MOV.

Enabling Playback Controls
Playback controls are essential for a good user experience. They allow users to play, pause, fast-forward, and rewind the video. Most video player libraries for React Native provide built-in playback controls that you can enable by setting a prop. For example, in react-native-video, you can enable controls by setting the 'controls' prop to true: 'controls={true}'. However, if you want more control over the look and feel of the playback controls, you can create custom controls. This involves creating a separate component for the controls and using the video player's methods to control playback. For instance, you can use the 'play' and 'pause' methods to start and stop the video, and the 'seek' method to jump to a specific time in the video.
Adding Style: Make it Look Good!
Finally, let's talk about styling the video player. A well-designed video player can greatly enhance the user interface of your app. You can style the video player by passing a style object to the 'style' prop. The style object can contain any valid React Native style properties. For example, you can set the width and height of the video player, change the background color, add padding and margins, and more. You can also style the playback controls if you're using custom controls. Remember, the goal is to create a video player that not only works well but also looks good and fits seamlessly into your app's design.
Popular React Native Video Players
React Native, a popular framework for building mobile applications, offers a variety of video player libraries that developers can leverage to integrate video playback functionality into their apps. These libraries provide a wide range of features, from basic video playback to advanced functionalities like live streaming, fullscreen mode, picture-in-picture playback, and subtitle support. One of the most popular libraries is the React Native Video library. This open-source project provides a comprehensive set of features, including support for various video formats, fullscreen playback, and customizable controls. It's easy to install and use, with detailed documentation and a supportive community of developers. Another widely used library is the React Native Video Player. This library offers a simple and intuitive API, making it easy for developers to integrate video playback into their apps. It supports a variety of video formats and provides features like fullscreen mode, picture-in-picture playback, and customizable controls. The React Native Media Player is another excellent option. This library provides a robust set of features, including support for live streaming, subtitle support, and customizable controls. It also offers a user-friendly API, making it easy for developers to integrate video playback into their apps. Lastly, the React Native Video Controls library is worth mentioning. This library provides a set of customizable controls for video playback, allowing developers to create a unique and intuitive user experience. It's easy to install and use, with detailed documentation and a supportive community of developers. In conclusion, these libraries offer a wide range of features and capabilities, making it easy for developers to integrate video playback into their React Native apps. They are easy to install and use, with detailed documentation and supportive communities of developers.
Advanced Features & Customization
Fullscreen Mode
Implementing fullscreen mode in a React Native video player is a straightforward process. Most libraries provide built-in support for this feature, allowing developers to enable fullscreen mode with just a few lines of code. For example, in the React Native Video library, developers can enable fullscreen mode by setting the 'fullscreen' prop to true. This will make the video player expand to fill the entire screen when the video is playing. In the React Native Video Player library, developers can enable fullscreen mode by calling the 'presentFullscreenPlayer' method. This will make the video player expand to fill the entire screen, providing an immersive viewing experience for the user. In the React Native Media Player library, developers can enable fullscreen mode by setting the 'fullscreen' prop to true. This will make the video player expand to fill the entire screen, providing an immersive viewing experience for the user. In conclusion, implementing fullscreen mode in a React Native video player is a straightforward process, with most libraries providing built-in support for this feature.
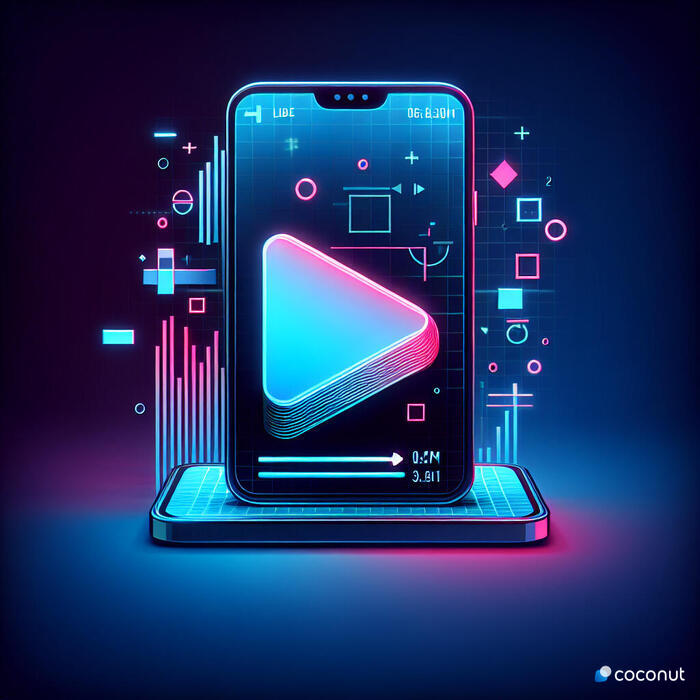
Picture-in-Picture (PiP) Playback
Picture-in-Picture (PiP) playback is a feature that allows users to watch a video in a small window while using other apps. This feature is supported by most React Native video player libraries, allowing developers to provide a seamless multitasking experience for their users. For example, in the React Native Video library, developers can enable PiP playback by setting the 'playInBackground' and 'playWhenInactive' props to true. This will allow the video to continue playing in a small window when the user switches to another app. In the React Native Video Player library, developers can enable PiP playback by setting the 'backgroundMode' prop to 'audio'. This will allow the video to continue playing in a small window when the user switches to another app. In the React Native Media Player library, developers can enable PiP playback by setting the 'backgroundMode' prop to 'audio'. This will allow the video to continue playing in a small window when the user switches to another app. In conclusion, PiP playback is a useful feature that allows users to multitask while watching a video. It's supported by most React Native video player libraries, making it easy for developers to provide this functionality in their apps.
Subtitle Support
Subtitles are an important feature for video players, as they enhance accessibility and allow users to understand the video content better. Most React Native video player libraries provide built-in support for subtitles, allowing developers to easily add this feature to their apps. For example, in the React Native Video library, developers can add subtitles to a video by setting the 'textTracks' prop. This prop accepts an array of objects, each representing a subtitle track. Each object should contain the type, language, and URL of the subtitle file. In the React Native Video Player library, developers can add subtitles to a video by setting the 'subtitle' prop. This prop accepts a string representing the URL of the subtitle file. In the React Native Media Player library, developers can add subtitles to a video by setting the 'subtitle' prop. This prop accepts a string representing the URL of the subtitle file. In conclusion, adding subtitles to a video is a straightforward process in React Native. Most libraries provide built-in support for this feature, making it easy for developers to enhance the accessibility of their video content.
Live Streaming
Live streaming is a popular feature in video players, allowing users to watch real-time video content. Most React Native video player libraries provide built-in support for live streaming, making it easy for developers to integrate this feature into their apps. For example, in the React Native Video library, developers can enable live streaming by setting the 'source' prop to the URL of the live stream. The library will then handle the streaming process, providing a seamless viewing experience for the user. In the React Native Video Player library, developers can enable live streaming by setting the 'source' prop to the URL of the live stream. The library will then handle the streaming process, providing a seamless viewing experience for the user. In the React Native Media Player library, developers can enable live streaming by setting the 'source' prop to the URL of the live stream. The library will then handle the streaming process, providing a seamless viewing experience for the user. In conclusion, live streaming is a popular feature in video players, and it's easy to implement in React Native. Most libraries provide built-in support for this feature, making it easy for developers to provide real-time video content in their apps.
Custom Controls and Overlays
Custom controls and overlays allow developers to create a unique and intuitive user experience in their video players. Most React Native video player libraries provide built-in support for custom controls and overlays, making it easy for developers to customize the look and feel of their video players. For example, in the React Native Video library, developers can create custom controls by using the 'control' prop. This prop accepts a React component, which will be rendered as the controls for the video player. Developers can create a custom React component with buttons for play/pause, rewind, fast forward, and other actions. In the React Native Video Player library, developers can create custom overlays by using the 'overlay' prop. This prop accepts a React component, which will be rendered as an overlay on top of the video player. Developers can create a custom React component with information about the video, such as the title, description, and progress bar. In the React Native Media Player library, developers can create custom controls and overlays by using the 'controls' and 'overlay' props. These props accept React components, which will be rendered as the controls and overlay for the video player. Developers can create custom React components with buttons for play/pause, rewind, fast forward, and other actions, as well as information about the video. In conclusion, custom controls and overlays are a powerful feature that allows developers to create a unique and intuitive user experience in their video players. Most React Native video player libraries provide built-in support for this feature, making it easy for developers to customize the look and feel of their video players.
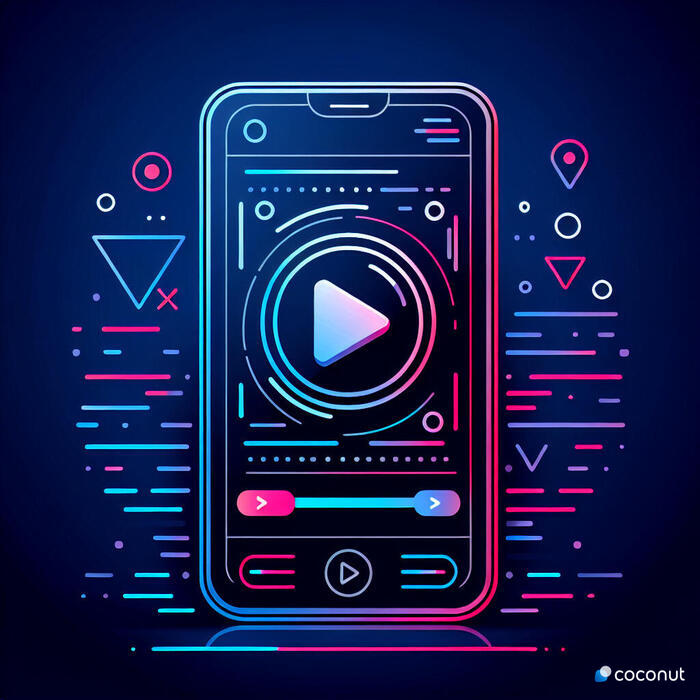
Why is React Native Video Player Popular?
React Native video player libraries are popular because they provide a comprehensive set of features and capabilities, making it easy for developers to integrate video playback into their apps. They are easy to install and use, with detailed documentation and supportive communities of developers. One of the main advantages of using video content in mobile apps is that it enhances user engagement. Videos are more engaging than text or images, and they can convey information in a more effective and immersive way. By integrating video playback into their apps, developers can provide a richer and more engaging user experience. React Native video player libraries also offer a high degree of customization. Developers can customize the look and feel of the video player, create custom controls and overlays, and add advanced features like fullscreen mode, picture-in-picture playback, and live streaming. This allows developers to create a unique and intuitive user experience, tailored to the needs and preferences of their users. In conclusion, React Native video player libraries are popular because they provide a comprehensive set of features and capabilities, a high degree of customization, and a supportive community of developers. By integrating video playback into their apps, developers can enhance user engagement, provide a richer user experience, and create a unique and intuitive user interface.
Optimizing React Native Video for Smooth Playback
In the realm of mobile application development, React Native has emerged as a game-changer. It's a framework that allows developers to build native apps using JavaScript and React. One of the many features that make React Native a popular choice among developers is its ability to handle video playback. However, to ensure a seamless user experience, it's crucial to optimize video playback in your React Native apps. The first step in optimizing video playback is choosing the right video format. React Native supports a wide range of video formats, including MP4, WebM, and Ogg. However, not all formats are created equal. Some formats offer better compression, which can lead to smoother playback and less data usage. Therefore, it's essential to choose a format that strikes a balance between quality and performance.Next, consider the size of the video files. Larger files take longer to load and can cause buffering issues, leading to a poor user experience. To avoid this, it's advisable to compress your video files without compromising too much on quality. There are several tools available online that can help you achieve this.Another crucial aspect of optimizing video playback in React Native is efficient buffering. Buffering is the process of pre-loading data into a reserved area of memory, so the video plays without interruption. You can optimize buffering by adjusting the buffer size based on the user's network conditions. For instance, if the user is on a slow network, you might want to increase the buffer size to prevent playback interruptions.Lastly, don't forget about error handling. Errors during video playback can lead to a frustrating user experience. Therefore, it's essential to implement robust error handling mechanisms in your React Native apps. This could involve displaying a user-friendly error message or automatically retrying the video playback after a certain period.By implementing these strategies, you can optimize video playback in your React Native apps and provide a seamless user experience.
Wrapping Up
Incorporating a video player into your React Native apps can significantly enhance user engagement. Videos are a powerful medium that can convey a lot of information in a short amount of time. They can be used to demonstrate how to use an app, showcase product features, or even provide entertainment. However, to reap the benefits of video content, it's crucial to ensure smooth video playback. By optimizing video formats, compressing video files, efficiently managing buffering, and handling errors effectively, you can provide a seamless video playback experience to your users. This not only enhances user engagement but also contributes to the overall success of your app.
FAQs
Supported Video Formats
React Native supports a wide range of video formats, including MP4, WebM, and Ogg. However, the compatibility of these formats can vary depending on the device and operating system. Therefore, it's advisable to test your videos on different devices and operating systems to ensure compatibility. If you encounter any issues, you might need to convert your videos to a different format. There are several online tools available that can help you with this.
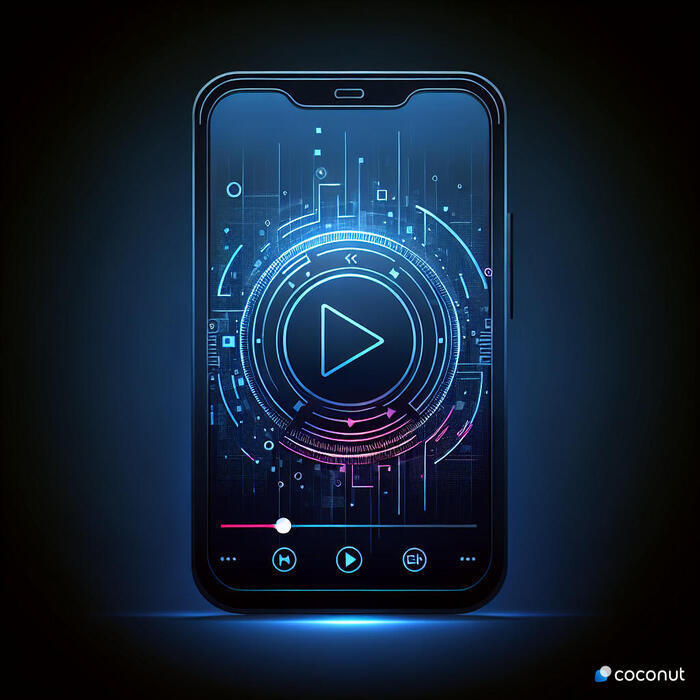
Error Handling
Errors during video playback can lead to a frustrating user experience. Therefore, it's essential to implement robust error handling mechanisms in your React Native apps. This could involve displaying a user-friendly error message or automatically retrying the video playback after a certain period. Additionally, you should also log these errors for further analysis. This can help you identify common issues and improve your app's video playback capabilities.
Live Streaming Implementation
Implementing live streaming in React Native can be a complex task, but it's definitely achievable. You'll need to use a streaming protocol, such as HLS or DASH, and a server that supports live streaming. Additionally, you'll need to handle various aspects like buffering, quality adjustment, and error handling. It's also advisable to test your live streams on different devices and network conditions to ensure a smooth user experience.
Playing YouTube Videos
Integrating YouTube videos into your React Native apps can be done using the YouTube API. You'll need to register your app with YouTube and obtain an API key. Once you have the API key, you can use it to fetch video data from YouTube and play it in your app. However, keep in mind that YouTube's terms of service restrict certain types of video playback, so make sure to review these before integrating YouTube videos into your app.